制作一个简单的数据库访问程序。(VC等)
附:以图书管理系统为例,图书管理系统经过数据需求分析得到的数据项借阅人信息:学号(stuid),姓名(name),性别(sex),科别代号(depno),科别名(depname),年级(grade),班级(class),电话(tel),
地址(addr)
书籍信息:书籍编号(code),书籍名称(bookname),作者(writer出版商(publisher),购买日期(buydate),借阅次数(number),是否借出(isloan),
借阅信息:借书人号、书号、借阅日期(loandate),归还日期(returndate)要求能完成下列事务处理要求
1.学生和图书信息的增、删、改及查询操作。
2.借书和还书操作。
其中图书管理员可以更新书库;图书操作人员可以进行借、还书操作;借书人可以查阅图书及自己借阅情况。
设计概述:
本系统语言采用c#实现,c#有很多可视化的预制件,直接拖动就可以了,只需要写很少的代码,规定数据来自于何处,如何修改,和筛选就可以了,先根据题目要求建立数据库并建立三个表。再设计四种权限操作,一是借阅管理员,实现对借阅记录信息的增删查改,设计2个窗口,一个窗口用来修改数据,一个窗口用来查询记录。二是图书管理员,实现对图书的增删查改,一个窗口就能实现。三是学生权限系统,实现查看自己的已借书信息,查询书库内容两个功能,分别设计成两个窗口。四是超级用户,可以实现对三个表的增删查改,在前面的基础上只需要创建登入的选择功能界面和学生表操作界面即可,再设计登录窗口,用来区别权限。
在做这个实验时有三本书对我提供了很大的帮助,是关于c#和.NET的书,书名我记不清了,已经过去大半年了。但是这种书应该都会介绍这个专门的数据库预制件还有c#的相关语法。
在进行编写代码之前首先要对环境进行配置。我使用的是sql server2019和vs2017.在vs2017中需要安装相应数据库的组件,然后再在c#工程中导入数据源。
1,建库并插入数据
建库的代码就不贴了,这里我直接是用数据库管理工具可视化建库的,下面的是数据初始化代码
insert into Booker_info values('1805120904','邓超越','男','1','智能科学',3,1,'123456','TS6-113')
insert into Booker_info values('1805121005','樊煦杰','男','2','计算机科学',3,1,'122456','TS6-113')
insert into Booker_info values('1805120921','孙天悦','男','1','智能科学',3,1,'122256','TS6-115')
insert into Booker_info values('1805120714','李玉','女','3','数字媒体',3,2,'133456','TS7-116')
insert into Booker_info values('1805120607','叶蕊秋','女','4','物联网',3,3,'124456','TS7-114')
insert into Book_info values('1','C程序设计','谭浩强','清华大学出版社','2019/11/12',40,1)
insert into Book_info values('2','数据库系统','王珊','高等教育出版社','2019/11/11',27,0)
insert into Book_info values('3','C++程序设计','郭炜','高等教育出版社','2019/10/13',44,0)
insert into Book_info values('4','操作系统','汤小丹','西安电子出版社','2019/08/07',31,1)
insert into Book_info values('5','数字图像处理','阮秋绮','电子工业出版社','2019/04/06',25,1)
insert into Lend_info values('1805120904','1','2020/11/12','2020/12/01')
insert into Lend_info values('1805121005','2','2020/11/10','2020/12/01')
insert into Lend_info values('1805120607','3','2020/11/09','2020/12/01')
insert into Lend_info values('1805120714','4','2020/11/07','2020/12/01')
insert into Lend_info values('1805120921','5','2020/11/11','2020/12/01')
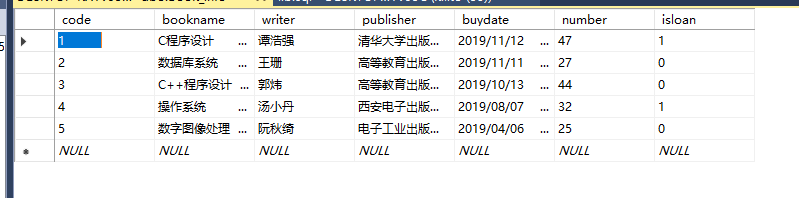

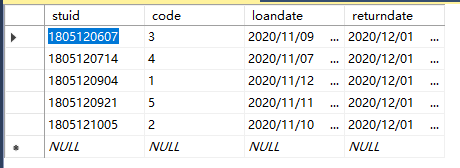
这是一个自动生成的Program.cs文件但是里面需要更改一些东西,指定入口窗口程序
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 系统
{
static class Program
{
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new 登录窗口());
}
}
}
2,创建登录界面,并分配权限
中国密码框可以选择是否隐藏输入的数据,类似于输入显示*号
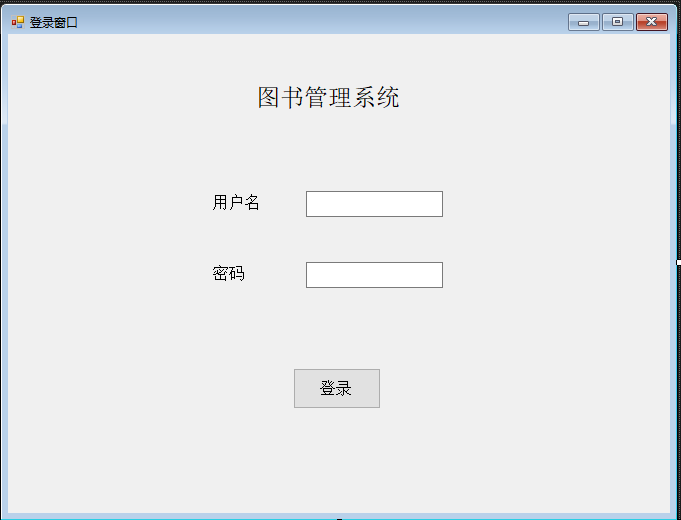
c#源码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 系统
{
public partial class 登录窗口 : Form
{
public 登录窗口()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
if (textBox2.Text.ToString() == "123456"&& textBox1.Text.ToString() =="jieyueadmin" )
{
name.sno = textBox1.Text.ToString();
借阅管理员 insert = new 借阅管理员();
insert.Show();
this.Hide();
}
else if (textBox2.Text.ToString() == "123456" && textBox1.Text.ToString() == "1805120904")
{
name.sno = textBox1.Text.ToString();
学生系统 insert = new 学生系统();
insert.Show();
this.Hide();
}
else if (textBox2.Text.ToString() == "123456" && textBox1.Text.ToString() == "libadmin")
{
name.sno = textBox1.Text.ToString();
图书管理员 insert = new 图书管理员();
insert.Show();
this.Hide();
}
else if (textBox2.Text.ToString() == "123456" && textBox1.Text.ToString() == "sysadmin")
{
name.sno = textBox1.Text.ToString();
系统管理员 insert = new 系统管理员();
insert.Show();
this.Hide();
}
else
MessageBox.Show("用户或密码错误!");
}
private void 登录窗口_Load(object sender, EventArgs e)
{
}
}
}
3,建立借阅管理员登录后界面,该用户可以对借阅记录实现查询和修改,以实现借阅功能
下面的数据显示c#有独立的预制件DataGridView,和QT类似,只需要拖动它到窗口中然后选择数据源就可以了。这个预制件十分强大,在预制件中可以设置选择是否有新增和编辑权限,甚至还有排序功能,唯独没有筛选功能需要我们自己写在后台代码中
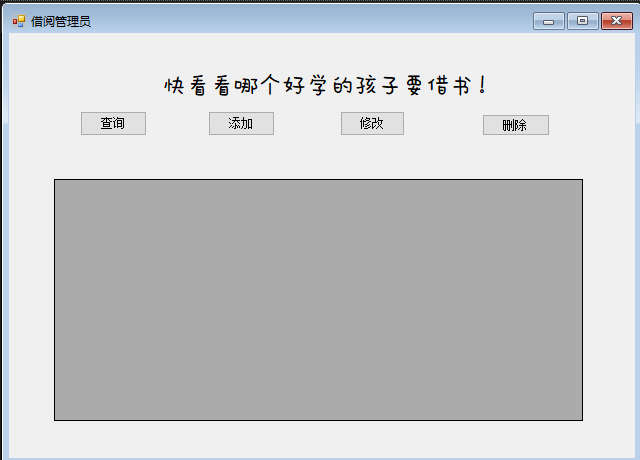
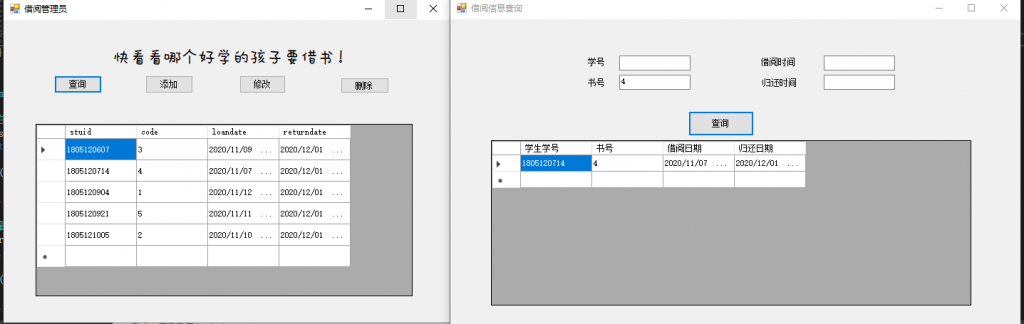
源码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace 系统
{
public partial class 借阅管理员 : Form
{
public 借阅管理员()
{
InitializeComponent();
}
private SqlConnection conn;
private SqlDataAdapter sqlada;
private SqlCommandBuilder sqlbd;
private DataSet ds;
private void Form1_Load(object sender, EventArgs e)
{
// TODO: 这行代码将数据加载到表“jXGLDataSet.STUDENT1”中。您可以根据需要移动或删除它。
this.sTUDENT1TableAdapter.Fill(this.jXGLDataSet.STUDENT1);
string connstr = "server = DESKTOP-KA1V95O;database=JXGL;Integrated Security=SSPI;Persist Security Info=false";
SqlConnection mysqlconnection = new SqlConnection(connstr);
mysqlconnection.Open();
string sqlstring = "select * from Lend_info";
conn = new SqlConnection(connstr);
conn.Open();
sqlada = new SqlDataAdapter(sqlstring, conn);
sqlbd = new SqlCommandBuilder(sqlada);
ds = new DataSet();
sqlada.Fill(ds, "Lend_info");
dataGridView1.DataSource = ds.Tables["Lend_info"];
}
private void button2_Click(object sender, EventArgs e)
{
借阅信息查询 insert = new 借阅信息查询();
insert.Owner = this;
insert.Show();
}
private void button3_Click(object sender, EventArgs e)
{
sqlada.Update(ds.Tables["Lend_info"]);
MessageBox.Show("更新完成!");
}
private void button1_Click(object sender, EventArgs e)
{
sqlada.Update(ds.Tables["Lend_info"]);
MessageBox.Show("更新完成!");
}
private void button4_Click(object sender, EventArgs e)
{
dataGridView1.Rows.Remove(dataGridView1.CurrentRow);
MessageBox.Show("单击修改按钮以完成删除");
}
private void label1_Click(object sender, EventArgs e)
{
}
}
}
4,建立图书管理员界面
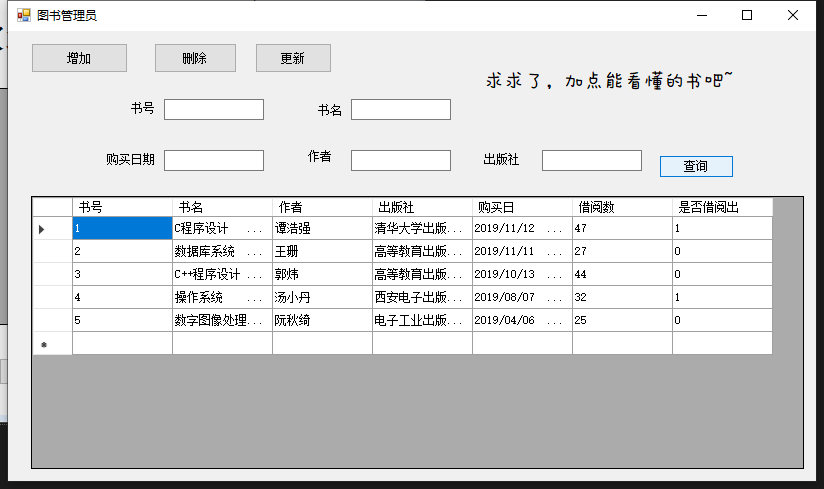
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace 系统
{
public partial class 图书管理员 : Form
{
//private SqlConnection conn;
// private SqlDataAdapter sqlada;
private SqlCommandBuilder sqlbd;
// private DataSet ds;
public 图书管理员()
{
InitializeComponent();
}
private void 图书管理员_Load(object sender, EventArgs e)
{
// TODO: 这行代码将数据加载到表“jXGLDataSet1.Book_info”中。您可以根据需要移动或删除它。
this.book_infoTableAdapter.Fill(this.jXGLDataSet1.Book_info);
/*
string connstr = "server = DESKTOP-KA1V95O;database=JXGL;Integrated Security=SSPI;Persist Security Info=false";
SqlConnection mysqlconnection = new SqlConnection(connstr);
mysqlconnection.Open();
string sqlstring = "select * from Book_info";
conn = new SqlConnection(connstr);
conn.Open();
sqlada = new SqlDataAdapter(sqlstring, conn);
sqlbd = new SqlCommandBuilder(sqlada);
ds = new DataSet();
sqlada.Fill(ds, "Book_info");
dataGridView1.DataSource = ds.Tables["Book_info"];
*/
}
private DataSet myDataSet;
private SqlConnection mySqlConnection;
private SqlDataAdapter mySqlDataAdapter;
private void button1_Click(object sender, EventArgs e)
{
mySqlDataAdapter.Update(myDataSet.Tables["Book_info"]);
MessageBox.Show("更新完成!");
}
private void button2_Click(object sender, EventArgs e)
{
dataGridView1.Rows.Remove(dataGridView1.CurrentRow);
MessageBox.Show("单击更新按钮以完成删除");
}
private void button3_Click(object sender, EventArgs e)
{
mySqlDataAdapter.Update(myDataSet.Tables["Book_info"]);
MessageBox.Show("更新完成!");
}
private void toolStripLabel1_Click(object sender, EventArgs e)
{
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
}
private void button4_Click(object sender, EventArgs e)
{
string connStr = "server = DESKTOP-KA1V95O;database = JXGL ;Integrated Security = SSPI;Persist Security Info = false";
//建立数据库新连接
mySqlConnection = new SqlConnection(connStr);
mySqlConnection.Open(); //打开数据库连接
//定义数据库查询字符串,采用通配符%
string cmdStr = "Select code as 书号,bookname as 书名,writer as 作者,publisher as 出版社,buydate as 购买日,number as 借阅数,isloan as 是否借阅出 from Book_info where " +
"code like '" + textBox1.Text.ToString() + "%' and " +
"bookname like '" + textBox2.Text.ToString() + "%' and " +
"writer like '" + textBox5.Text.ToString() + "%' and " +
"buydate like '" + textBox4.Text.ToString() + "%' and " +
"publisher like '" + textBox3.Text.ToString() + "%' ";
mySqlDataAdapter = new SqlDataAdapter(cmdStr, mySqlConnection);
sqlbd = new SqlCommandBuilder(mySqlDataAdapter);
//创建一个空的数据集对象
myDataSet = new DataSet();
//调用SqlDataAdapter对象的fill方法时,查询数据库,将结果填入DataSet
mySqlDataAdapter.Fill(myDataSet, "Book_info");
//把Dataset的数据赋给DataSource
dataGridView1.DataSource = myDataSet.Tables["Book_info"];
mySqlConnection.Close();
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
}
}
5,建立学生权限系统
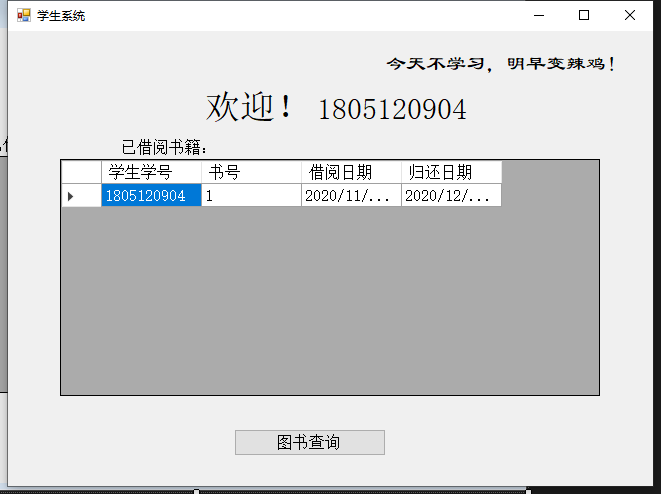
该界面可以实现查看自己所借阅的书籍
点击图书查询后,可实现对图书目录的检索,但不具备修改权限
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace 系统
{
public partial class 学生系统 : Form
{
public 学生系统()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void 学生系统_Load(object sender, EventArgs e)
{
label3.Text = name.sno;
//定义数据库查询字符串,采用通配符%
string connStr = "server = DESKTOP-KA1V95O;database = JXGL ;Integrated Security = SSPI;Persist Security Info = false";
//建立数据库新连接
SqlConnection mySqlConnection = new SqlConnection(connStr);
mySqlConnection.Open(); //打开数据库连接
//定义数据库查询字符串,采用通配符%
string cmdStr = "Select stuid as 学生学号,code as 书号,loandate as 借阅日期,returndate as 归还日期 from Lend_info where stuid ="+name.sno;
SqlDataAdapter mySqlDataAdapter = new SqlDataAdapter(cmdStr, mySqlConnection);
//创建一个空的数据集对象
DataSet myDataSet = new DataSet();
//调用SqlDataAdapter对象的fill方法时,查询数据库,将结果填入DataSet
mySqlDataAdapter.Fill(myDataSet, "Lend_info");
//把Dataset的数据赋给DataSource
dataGridView1.DataSource = myDataSet.Tables["Lend_info"];
mySqlConnection.Close();
dataGridView1.AllowUserToAddRows = false;
dataGridView1.AllowUserToDeleteRows = false;
}
private void label3_Click(object sender, EventArgs e)
{
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
学生图书查询 insert = new 学生图书查询();
insert.Owner = this;
insert.Show();
}
}
}
点击图书查询后,可实现对图书目录的检索,没有修改权限
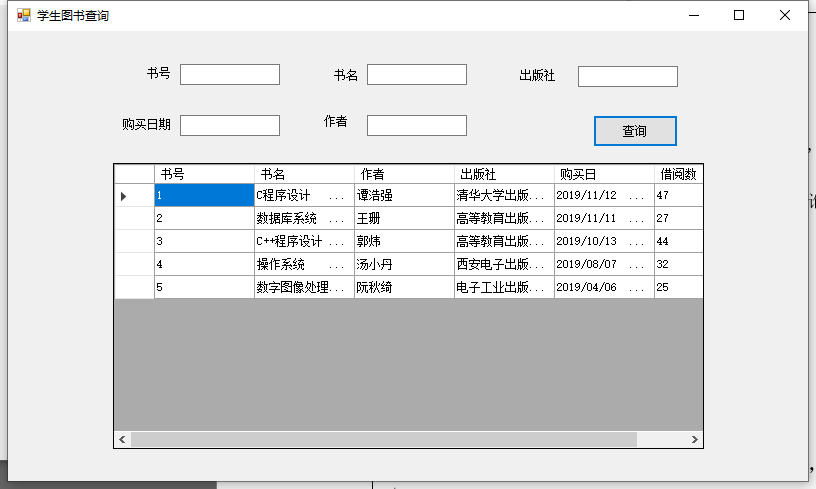
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace 系统
{
public partial class 学生图书查询 : Form
{
public 学生图书查询()
{
InitializeComponent();
}
private void 学生图书查询_Load(object sender, EventArgs e)
{
}
private void label4_Click(object sender, EventArgs e)
{
}
private void label5_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
string connStr = "server = DESKTOP-KA1V95O;database = JXGL ;Integrated Security = SSPI;Persist Security Info = false";
//建立数据库新连接
SqlConnection mySqlConnection = new SqlConnection(connStr);
mySqlConnection.Open(); //打开数据库连接
//定义数据库查询字符串,采用通配符%
string cmdStr = "Select code as 书号,bookname as 书名,writer as 作者,publisher as 出版社,buydate as 购买日,number as 借阅数 from Book_info where " +
"code like '" + textBox1.Text.ToString() + "%' and " +
"bookname like '" + textBox2.Text.ToString() + "%' and " +
"writer like '" + textBox5.Text.ToString() + "%' and " +
"buydate like '" + textBox4.Text.ToString() + "%' and " +
"publisher like '" + textBox3.Text.ToString() + "%' ";
SqlDataAdapter mySqlDataAdapter = new SqlDataAdapter(cmdStr, mySqlConnection);
//创建一个空的数据集对象
DataSet myDataSet = new DataSet();
//调用SqlDataAdapter对象的fill方法时,查询数据库,将结果填入DataSet
mySqlDataAdapter.Fill(myDataSet, "Book_info");
//把Dataset的数据赋给DataSource
dataGridView1.DataSource = myDataSet.Tables["Book_info"];
mySqlConnection.Close();
dataGridView1.AllowUserToAddRows = false;
dataGridView1.AllowUserToDeleteRows = false;
}
private void textBox5_TextChanged(object sender, EventArgs e)
{
}
private void textBox4_TextChanged(object sender, EventArgs e)
{
}
private void textBox3_TextChanged(object sender, EventArgs e)
{
}
}
}
6,建立系统管理权限系统,即超级用户
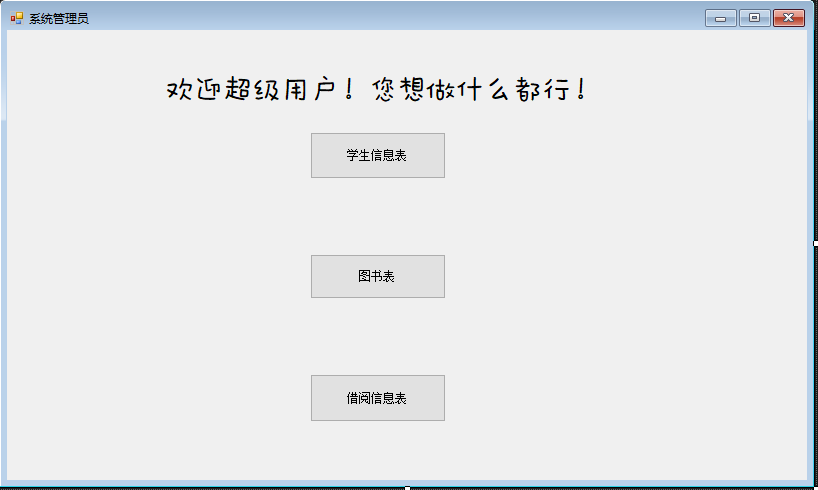
超级用户可对三个表都能进行增删查改的操作。其中图书表和借阅表直接用的是图书管理员权限界面,借阅表用的是借阅管理员权限界面,只增加了对学生表的操作。学生表操作界面如下图所示
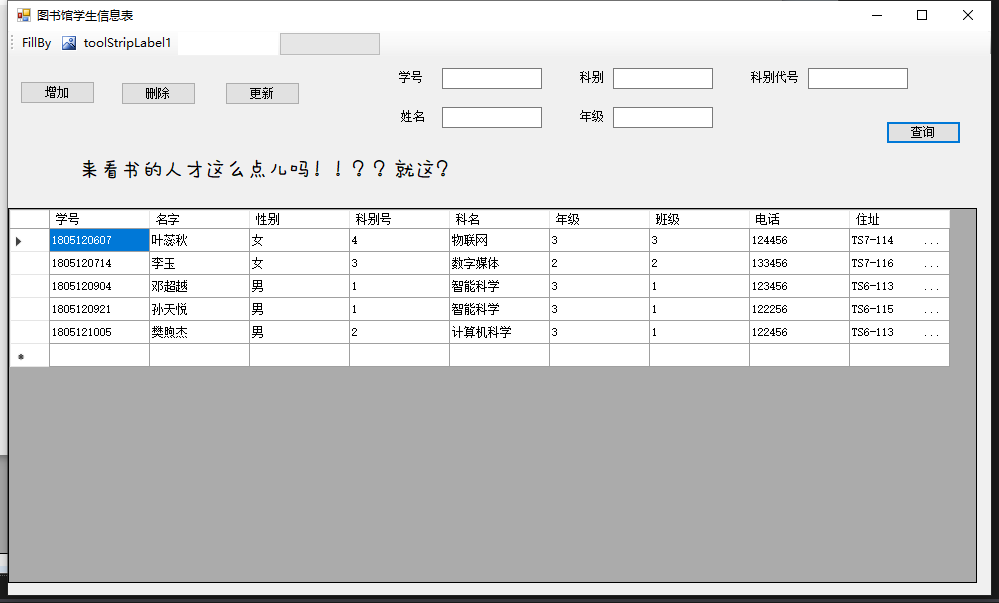
系统管理员登入界面c#源代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 系统
{
public partial class 系统管理员 : Form
{
public 系统管理员()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
图书馆学生信息表 insert = new 图书馆学生信息表();
insert.Owner = this;
insert.Show();
}
private void button2_Click(object sender, EventArgs e)
{
图书管理员 insert = new 图书管理员();
insert.Owner = this;
insert.Show();
}
private void button3_Click(object sender, EventArgs e)
{
借阅管理员 insert = new 借阅管理员();
insert.Owner = this;
insert.Show();
}
private void 系统管理员_Load(object sender, EventArgs e)
{
}
}
}
学生信息表界面c#代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace 系统
{
public partial class 图书馆学生信息表 : Form
{
// private SqlConnection conn;
//private SqlDataAdapter sqlada;
private SqlCommandBuilder sqlbd;
// private DataSet ds;
public 图书馆学生信息表()
{
InitializeComponent();
}
private void 图书馆学生信息表_Load(object sender, EventArgs e)
{
// TODO: 这行代码将数据加载到表“jXGLDataSet1.Booker_info”中。您可以根据需要移动或删除它。
this.booker_infoTableAdapter.Fill(this.jXGLDataSet1.Booker_info);
/* string connstr = "server = DESKTOP-KA1V95O;database=JXGL;Integrated Security=SSPI;Persist Security Info=false";
SqlConnection mysqlconnection = new SqlConnection(connstr);
mysqlconnection.Open();
string sqlstring = "select * from Booker_info";
conn = new SqlConnection(connstr);
conn.Open();
sqlada = new SqlDataAdapter(sqlstring, conn);
sqlbd = new SqlCommandBuilder(sqlada);
ds = new DataSet();
sqlada.Fill(ds, "Booker_info");
dataGridView1.DataSource = ds.Tables["Booker_info"];
*/
}
private DataSet myDataSet;
private SqlConnection mySqlConnection;
private SqlDataAdapter mySqlDataAdapter;
private void fillByToolStripButton_Click(object sender, EventArgs e)
{
try
{
this.booker_infoTableAdapter.FillBy(this.jXGLDataSet1.Booker_info);
}
catch (System.Exception ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message);
}
}
private void button1_Click(object sender, EventArgs e)
{
mySqlDataAdapter.Update(myDataSet.Tables["Booker_info"]);
MessageBox.Show("更新完成!");
}
private void button4_Click(object sender, EventArgs e)
{
mySqlDataAdapter.Update(myDataSet.Tables["Booker_info"]);
MessageBox.Show("更新完成!");
}
private void button2_Click(object sender, EventArgs e)
{
dataGridView1.Rows.Remove(dataGridView1.CurrentRow);
MessageBox.Show("单击更新按钮以完成删除");
}
private void button3_Click(object sender, EventArgs e)
{
string connStr = "server = DESKTOP-KA1V95O;database = JXGL ;Integrated Security = SSPI;Persist Security Info = false";
//建立数据库新连接
mySqlConnection = new SqlConnection(connStr);
mySqlConnection.Open(); //打开数据库连接
//定义数据库查询字符串,采用通配符%
string cmdStr = "Select stuid as 学号,name as 名字,sex as 性别,depno as 科别号,depname as 科名,grade as 年级,class as 班级,tel as 电话,addr as 住址 from Booker_info where " +
"stuid like '" + textBox1.Text.ToString() + "%' and " +
"name like '" + textBox2.Text.ToString() + "%' and " +
"depno like '" + textBox5.Text.ToString() + "%' and " +
"grade like '" + textBox4.Text.ToString() + "%' and " +
"depname like '" + textBox3.Text.ToString() + "%' ";
mySqlDataAdapter = new SqlDataAdapter(cmdStr, mySqlConnection);
sqlbd = new SqlCommandBuilder(mySqlDataAdapter);
//创建一个空的数据集对象
myDataSet = new DataSet();
//调用SqlDataAdapter对象的fill方法时,查询数据库,将结果填入DataSet
mySqlDataAdapter.Fill(myDataSet, "Booker_info");
//把Dataset的数据赋给DataSource
dataGridView1.DataSource = myDataSet.Tables["Booker_info"];
mySqlConnection.Close();
}
private void label3_Click(object sender, EventArgs e)
{
}
private void fillByToolStrip_ItemClicked(object sender, ToolStripItemClickedEventArgs e)
{
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
}
private void textBox2_TextChanged(object sender, EventArgs e)
{
}
private void textBox3_TextChanged(object sender, EventArgs e)
{
}
private void textBox4_TextChanged(object sender, EventArgs e)
{
}
private void textBox5_TextChanged(object sender, EventArgs e)
{
}
}
}
通过这次实验,大致了解了数据库和高级语言的链接以及数据的传递。切实感受到了数据库系统对软件开发所带来的便利。之前设计的图书管理系统由于没有用数据库,需要进行繁琐的文件存储操作和格式控制以及输入输出控制,开发过程十分复杂。但数据哭系统为开发软件的数据源提供了很好的整合,在软件的开发种注重功能的实现,而不用过于操心数据的连通,存储问题。 这次实验课使我有了初步开发软件的经验,用c#语言开发了一款十分简洁的图书管理系统,而在这之前没有任何图形界面软件的开发经验,也没有c#语言基础。但凭借着对c++语言的熟悉,还是很快就上手了这门语言。这让我认识到,在项目和任务中学习,会使学习效率提高不少,带着任务和问题看书,才能在解决问题中真正掌握技能。
1 条评论
fast loto casino · 2023年4月20日 上午5:02
Thanks for providing information